According to the Python Developers Survey, Django ranks as the second most popular Python framework worldwide. Its comprehensive feature set, scalability, and robust security make it a compelling choice for building powerful and reliable business applications.
However, getting the most out of Django isn’t always straightforward. In this article, we’ll guide you through seven easy steps to use Django for business software development, from defining clear business requirements to testing and deploying your application. By following these steps, you can leverage Django’s strengths to create effective, scalable, and secure business solutions. Let’s jump into it!
Why do businesses choose the Django framework?
As a high-level Python-based web development framework, Django is popular for business application development for a few key reasons:
- Ease of use and simplicity: Django is known for its simplicity and ease of use, making it an excellent choice for both beginners and experienced developers. Its straightforward syntax and extensive documentation facilitate a smooth learning curve and efficient development process.
- Mature and stable framework: Django has been in use for over a decade, benefiting from a large supportive community and continuous improvements. Its maturity ensures stability and reliability, making it a trusted choice for many businesses.
- Comprehensive feature set: Many teams choose Django because it provides a wide range of features, including content administration, a templating system, and an object-relational mapper. Its "batteries included" philosophy means it comes with all the necessary components for rapid web app development right out of the box.
- High scalability: With Django, businesses can build highly scalable Django apps that can easily expand in features or resources as needed. This scalability makes it suitable for both smaller companies and complex corporate environments.
- Built-in security: Django offers built-in security features to protect against common web vulnerabilities such as SQL injection, cross-site scripting, and clickjacking.
7 easy steps to use Django for business software development
It’s clear that Django can offer some compelling benefits for teams looking to build business web applications. Now let’s dive into some of the more practical aspects of leveraging Django for your next project. In this section we’ll walk through 7 easy steps to guide you through the process:
Define clear business requirements
Before diving into development, it's crucial to define clear business requirements. Understanding your business's needs is the foundation of successful software development. Are you trying to optimize a specific business process? Offload your team from tedious or manual work? Prepare your business to scale?
To help you gain clarity, begin the formal requirements gathering process of collecting, organizing and documenting all functional and non-functional requirements of the proposed software. This process can be led by a project lead or project manager.
It is important at this stage to ensure that the requirements are not met, or could be closely met, by existing applications. The key output of this phase will be a business requirements document which should contain a list of all required functionality, possibly prioritized using a technique such as MoSCoW (Must have/Should have/Could have/Won’t have now).
Producing a requirements document as part of the Django software development process can help to:
- Verify that there is a legitimate need for the new software to be built rather than simply purchasing a software solution off the shelf.
- Ensure the software development team has complete clarity on the requirements of the proposed business software.
- Provide a high degree of transparency right from the beginning of a project to all stakeholders who may be involved.
- Prevent the final software from needing extensive rework or changes due to missing criteria.
- Validate that the capabilities being delivered by the new software meet the stated needs of the enterprise software project team.
Set up the Django development environment
Setting up your Django development environment correctly is crucial for a smooth and efficient development process. A well-configured environment ensures that your team can work effectively and helps prevent issues as you progress. Generally you’ll want to follow these key steps to get set up:
Install Python and Django
Since Django is a Python-based framework, the first step is to install Python. Ensure you have the latest version of Python installed on your system. Once Python is installed, you can proceed to install Django using Python's package manager, pip. This can be done with the command pip install django.
Set up a virtual environment
Using virtual environments is a best practice for Python development. A virtual environment isolates your project dependencies from the system-wide packages, preventing conflicts between different projects. You can create a virtual environment using the venv module included with Python or tools like virtualenv. Activate the virtual environment before installing any project dependencies to ensure they are contained within the environment.
This could look something like:
python -m venv .venv
source .venv/bin/activate
pip install django
Start a new project
You can start a new Django project using the django-admin command. This will set up the basic structure of your django application.
Let’s look at an example of this process:
django-admin startproject my_django_project
Within the project you can create multiple apps, where each one handles a specific part of the project’s functionality. Use manage.py script to create a new app.
python manage.py startapp myapp
New apps should be added to settings.py file. This will ensure that Django recognizes your app and includes it in the project.
INSTALLED_APPS = [
...
'myapp', # Add your app here
]
Configure database
Django supports several database backends, including PostgreSQL, MySQL, and SQLite. For development purposes, SQLite is often sufficient as it requires no additional setup. However, for production environments, it's recommended to use a more robust database like PostgreSQL or MySQL. Configure your database settings in the Django project's settings file to ensure proper connectivity and performance.
Example postgres configuration:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': 'mydatabase',
'USER': 'mydatabaseuser',
'PASSWORD': 'mypassword',
'HOST': 'localhost',
'PORT': '5432',
}
}
After setting up the project, run the initial migrations to create necessary database tables.
python manage.py migrate
python manage.py makemigrations
Finally, start the development server using python manage.py runserver.
# Use an official Python runtime as a parent image FROM python:3.9-slim # Set the working directory WORKDIR /usr/src/app # Install dependencies COPY requirements.txt ./ RUN pip install --no-cache-dir -r requirements.txt # Copy the project files COPY . . # Run the application CMD ["python", "manage.py", "runserver", "0.0.0.0:8000"]
Design your data model
Designing your business model is a critical step in developing a Django application that meets your business needs. A well-planned database structure and thoughtfully defined models ensure that your application can handle data efficiently and effectively. Designing your model breaks down into two key tasks:
Plan the database structure
Start by planning the database structure to fit your business requirements. (The more work you’ve put into Step #1, the easier this will be!) Understand the data you need to store, the relationships between different data entities, and how the data will be accessed and manipulated. Create a detailed schema outlining all the tables, fields, and relationships. This planning phase is crucial for ensuring that your database can scale and adapt as your business grows.
Define models in Django
In Django, models are used to represent your data and its structure. Each model corresponds to a table in the database, and each attribute of the model corresponds to a column in the table. Define your models in Django by creating classes that inherit from django.db.models.Model. These classes should include all the necessary fields and their respective data types. Defining models accurately ensures that your database operations are efficient and your data integrity is maintained.
When defining models in Django, using the repository pattern can help separate the business logic from data access logic, promoting a cleaner and more modular codebase.
Let’s look at an example:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
price = models.DecimalField(max_digits=10, decimal_places=2)
description = models.TextField()
stock = models.IntegerField()
created_at = models.DateTimeField(auto_now_add=True)
updated_at = models.DateTimeField(auto_now=True)
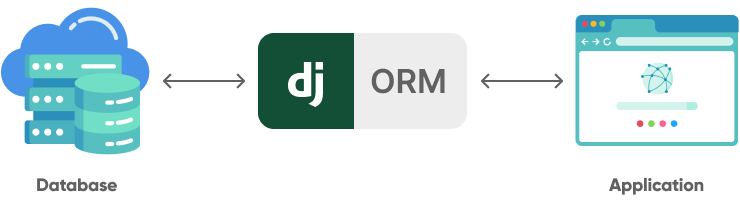
Build the business logic
Building the business logic is where you define how your application operates and handles specific business processes. In Django code, this involves writing custom views and templates to manage the flow of data and user interactions. Here’s how you can effectively build the business logic for your application:
Write custom views
Views in Django are Python specific functions or classes that handle requests and return responses. They are the heart of your application’s business logic. Custom views process user input, interact with the database, and determine what data to display. You can also build views to integrate and manage RSS feeds, providing dynamic content updates to your users.
Start by defining views that correspond to the key functionalities of your application. Use Django’s view system to handle different types of requests (GET, POST) and perform necessary actions. Django provides out-of-the-box solutions for common view types called generic views, which can speed up the development process.
These generic views encapsulate common patterns for rendering lists of objects, detailed views of single objects, and forms for creating new objects. Generic views are highly customisable, by overriding built in methods like get_queryset() or get_context_data().
Let’s look at an example:
from django.urls import reverse_lazy
from django.views.generic import ListView, DetailView, CreateView
from .models import Product
from .forms import ProductForm
class ProductListView(ListView):
model = Product
template_name = 'product_list.html'
context_object_name = 'products'
class ProductDetailView(DetailView):
model = Product
template_name = 'product_detail.html'
context_object_name = 'product'
class AddProductView(CreateView):
model = Product
form_class = ProductForm
template_name = 'add_product.html'
success_url = reverse_lazy('product_list')
In some cases, view logic may be complex and does not fit well within generic view patterns. In those cases, you can use the View class and define the request handling logic yourself. This provides more control and flexibility.
For example:
from django.shortcuts import render
from django.views import View
from .models import Product
class ProductListView(View):
def get(self, request):
products = Product.objects.all()
return render(request, 'product_list.html', {'products': products})
Create templates
Templates in the Django web framework are used to generate the HTML pages that are sent to the user’s browser. They define the structure of your web pages and are populated with dynamic data from the views. Use Django’s templating language to create reusable templates that can include logic for displaying data, iterating over items, and handling user inputs. This separation of concerns keeps your code clean and manageable.
Let’s build on the example above to create templates:
<!-- product_list.html -->
{% extends 'base.html' %}
{% block content %}
<h1>Product List</h1>
<ul>
{% for product in products %}
<li><a href="{% url 'product_detail' product.id %}">{{ product.name }}</a></li>
{% endfor %}
</ul>
{% endblock %}
Connect views and templates
Now, connect your views and templates by mapping URLs to views in Django’s URL configuration. This ensures that when users navigate to different parts of your application, the appropriate views are called and the correct templates are rendered. Define URL patterns in the urls.py file to create a clear and logical routing system.
This could look like:
from django.urls import path
from . import views
urlpatterns = [
path('products/', views.product_list, name='product_list'),
path('products/<int:product_id>/', views.product_detail, name='product_detail'),
path('products/add/', views.add_product, name='add_product'),
]
Create user interfaces
While the Django framework is primarily for backend web development, it can effectively work with frontend technologies through Django REST Framework (DRF) to build APIs that interact with libraries like React. This allows for a seamless integration of Django's powerful backend capabilities with dynamic, responsive user interfaces.
When creating UIs for business software development using Django, it's crucial to ensure that your interfaces are not only functional but also aesthetically pleasing and user-friendly. A well-designed UI can significantly enhance the user experience, making the software more intuitive and efficient for business users. Here are some key strategies to consider:
- Utilize front-end frameworks like Bootstrap or Foundation to streamline the design process and maintain a clean, professional look.
- Incorporate Django’s template inheritance to ensure consistency across your application by defining a base template for common elements such as the header, footer, and navigation bar.
- Enhance user interactions with JavaScript to add dynamic features and provide immediate feedback, making your application more engaging.
- Prioritize accessibility by following web accessibility standards (WCAG) to ensure that your UI is usable by everyone, including those with disabilities.
- Enhance your application's navigation by incorporating site maps. Site maps improve user experience by providing a clear structure of the website, making it easier for users to find the information they need.
Read More: UI Design Process - 7 Easy Steps to Make Great UIs Faster
Integrate third-party packages
Integrating third-party apps and services into your Django application can significantly enhance its functionality and provide a better user experience. By leveraging external tools and services, you can add features without having to build everything from scratch. Such solutions not only save development time but also ensure that you are using reliable and tested packages.
A few examples of third party packages to consider include:
- Django Guardian: Managing fine-grained permissions programmatically or via the Django admin can be tedious. Django Guardian provides a straightforward API to manage per-object user and group-level permissions efficiently, simplifying complex permission structures with minimal code. Consider organizing such functionalities into a separate module to keep your codebase modular and maintainable.
- Celery: Celery is a powerful task queue that allows you to schedule and run time-consuming, unpredictable, or resource-intensive processes outside the usual HTTP request/response cycle. Use Celery to automate tasks such as sending emails, processing large files, scraping web data, handling third-party API calls, analyzing data, and generating reports. It’s important to know that setting up Celery requires a message broker like Redis or RabbitMQ.
- Wagtail CMS: Wagtail CMS offers a highly customizable content management system that leverages Django’s capabilities to build content-rich websites. It provides robust page models and a flexible interface, making it an excellent choice for projects requiring advanced content management without the need for a custom-built CMS.
- Django Filter: Simplify the process of creating filters for your views with Django Filter. This package helps you avoid writing repetitive code for common query types, allowing users to request data based on parameters that map to database fields or attributes effortlessly.
- Sentry: Sentry is an open-source solution for monitoring website activity in production. It offers advanced logging, tracing, and error monitoring features through a simple interface. Sentry’s generous free tier makes it ideal for individual developers and small teams looking to avoid production issues and maintain application reliability.
Test and deploy business application
At the final stages of web development, thorough testing and careful deployment are critical to ensure your Django application is robust, reliable, and ready for real-world use.
End-to-end testing
A well-rounded testing strategy includes various types of tests to cover all aspects of the application, from individual components to overall system performance and security. Here are the key types of testing you should incorporate:
- Unit testing: Focus on individual components like models, views, and forms to ensure each part functions correctly in isolation. Django’s built-in testing framework facilitates writing these tests.
- Integration testing: Verify that different components of the application work together seamlessly. This ensures that models, views, and templates interact as intended.
- Functional testing: Evaluate the application from the user's perspective by simulating actions such as form submissions and page navigation. This type of testing ensures that the system works as a whole.
- End-to-end (E2E) testing: Cover complete user workflows to confirm that the application operates correctly in real-world scenarios, from user authentication to transaction processing.
- Performance testing: Assess the application's performance under load to identify bottlenecks and ensure it can handle high traffic and large volumes of data.
- Security testing: When configured correctly, Django will handle the protection against common vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). If needed, you can set up separate audit / testing scenarios to ensure this protection is in place.
Deployment
Once testing is complete, the deployment phase ensures your Django application is ready for production use. Proper deployment involves:
- Scaling for high traffic: Plan for scaling your application to handle increased traffic. This involves optimizing database queries, using caching strategies, and considering load balancing.
- Web server configuration: Configure your web server (e.g., Nginx, Apache) to serve your application efficiently. Proper configuration can improve performance and security.
- Choosing a hosting provider: Select a hosting provider that meets your needs. Options like AWS, Heroku, and DigitalOcean offer various features and pricing plans to support different requirements.
- Automating repetitive tasks: Automating repetitive tasks with custom management commands can significantly streamline the testing and deployment process, ensuring that your application remains robust and reliable.
Top challenges businesses face when using Django web framework
As you embark on your own Django project, it’s important to be aware of the common challenges that teams face with this web framework. Understanding these challenges can help you effectively manage and mitigate potential issues during development and deployment:
- Steep learning curve: Django's comprehensive set of features and conventions can be daunting for new developers. Understanding Django’s ORM, middleware, and the Model-Template-View (MTV) architecture takes time, potentially slowing initial development.
- Performance optimization: Django’s rich feature set can lead to performance overhead. Businesses must optimize their applications by fine-tuning database queries, implementing caching strategies, and writing efficient code to avoid slower response times and reduced responsiveness.
- Scalability concerns: While Django is scalable, preparing an application to handle significant traffic requires careful planning. This includes proper database design, load balancing, and using tools like Redis for caching and Celery for asynchronous tasks to prevent performance bottlenecks.
- Complex migrations: As applications evolve, database schema changes are inevitable. Django’s migration framework manages these changes, but complex migrations can be challenging, especially with large databases, requiring meticulous planning to ensure data integrity and minimize downtime.
- Integration with other systems: Integrating Django applications with legacy systems, third-party APIs, or other microservices can be complex and time-consuming. This requires thorough planning, robust error handling, and sometimes significant customization to ensure smooth communication between systems.
Conclusion
By following the seven steps outlined in this guide, you can leverage Django’s strengths to create scalable, secure, and efficient applications tailored to your business needs. With careful planning, strategic use of third-party services, and thorough testing, Django can help you build reliable and high-performing software solutions that drive business success for the long-term.
If you're looking for a Python Development Company to help you navigate these challenges, we’d love to help. Our business software development team will partner with you to build a comprehensive strategy to meet your business needs and deliver a user-ready, Django software solution.