The average employee spends nearly 5 hours a week creating PowerPoint decks. If you’re looking for ways to automate routine PowerPoint tasks, you’re not alone. Leveraging Python to build simple automation scripts can drastically reduce the monotonous work involved in creating and modifying PowerPoints.
In this article, we’ll look at 7 ways to boost productivity with Python’s PowerPoint automation libraries. By the end, you'll be able to use Python to create, manipulate, and enhance PowerPoint slides like a pro!
Get started automating PowerPoint with Python
PowerPoint automation with Python starts by choosing an appropriate library. Unlike Excel automation or Word automation, which have a variety of library options, there’s really only one major Python PowerPoint library: python-pptx.
Python-pptx is an open source Python library, which offers users a range of features including:
- Variety of pre-built layouts along with the ability to create custom layouts
- Automated tool to add images, audio and video files using a Python script
- Support for the addition and modification of charts, shapes, and tables
- Ability to handle only the .pptx format. You would need other libraries if you need to work with older PowerPoint formats (.ppt).
To start using python-pptx, you simply need to install it via pip and it is ready for use!
7 ways to boost productivity with PowerPoint automation
Automation of PowerPoint presentations using Python provides a powerful tool for reducing time and effort spent on slide creation and editing. In this section, we explore 7 practical methods to leverage Python's capabilities to automate and streamline your PowerPoint workflows, allowing your team to focus more on content quality and delivery.
Create PowerPoint presentations
The most basic of all automation tasks is to create PowerPoint presentations. There are two main ways to do this:
Method 1: Create PowerPoint presentations from scratch with Python
Pros | Cons |
---|---|
|
|
Method 2: Create PowerPoint presentations from templates
Pros | Cons |
---|---|
|
|
Modify PowerPoint slides
Python libraries are not just useful for PowerPoint deck creation; they can also be used for updating PowerPoint decks. With Python code, you can edit existing PowerPoint slides, add new slides or delete slides, change slide format, automate repetitive slides, and apply design styles to your presentations. Let’s look at an example.
Example 1: Make content changes to an existing slide deck
from pptx import Presentation
from pptx.util import Inches
from pptx.enum.shapes import MSO_SHAPE
# Load an existing presentation
ppt = Presentation('template.pptx')
# Add a new slide with a title and content layout
slide_layout = ppt.slide_layouts[1] # Title and Content layout
new_slide = ppt.slides.add_slide(slide_layout)
new_slide.shapes.title.text = "Added Slide"
new_slide.placeholders[1].text = "Content of the new slide."
# Modify text in the first existing slide
if ppt.slides:
first_slide = ppt.slides[0]
if first_slide.shapes.title:
first_slide.shapes.title.text = "Modified Title"
# Add a rectangle shape
left = Inches(2)
top = Inches(2)
width = Inches(3)
height = Inches(1)
shape = first_slide.shapes.add_shape(MSO_SHAPE.RECTANGLE, left, top, width, height)
shape.text = "Added Rectangle"
# Check if there is more than one slide to avoid errors
if len(ppt.slides) > 1:
slide_ids = ppt.slides._sldIdLst
slide_ids.remove(slide_ids[1]) # This removes the second slide
# Save the modified presentation
ppt.save('modified_presentation.pptx')
PRO TIP: To effectively modify PowerPoint slides, it can be helpful to understand the object structure of PowerPoint documents:
- The Presentation object represents the PowerPoint file.
- Each presentation can contain multiple slides (a title slide or traditional slides) which are represented by the Slide object.
- Individual elements such as shapes, tables, charts, and text boxes can be manipulated in Python.
Embed other documents into a PowerPoint presentation
Python allows for the embedding of several document types into PowerPoint presentations, including Word documents, Excel spreadsheets, images, videos, PDF files, and other PowerPoint presentations. Each document is encapsulated within its own OLE object shape. It’s worth noting that this feature requires the installation of the pywin32 library on a Windows machine.
Example 1: Embed an Excel file into PowerPoint slides
import os
import win32com.client
from pptx import Presentation
from pptx.util import Inches
# Paths and settings
excel_file_path = spreadsheet.xlsx'
ppt_template_path = template.pptx'
output_ppt_path = 'output_presentation.pptx'
# Start an instance of PowerPoint
app = win32com.client.Dispatch("PowerPoint.Application")
app.Visible = 1
# Open an existing PowerPoint file or create a new one
if os.path.exists(ppt_template_path):
presentation = app.Presentations.Open(ppt_template_path)
else:
presentation = app.Presentations.Add()
# Add a new slide at the end of the presentation
slide = presentation.Slides.Add(Index=presentation.Slides.Count + 1, Layout=12) # Layout 12 is typically a blank slide
# Define position and size for the OLE object
left = Inches(1)
top = Inches(1)
width = Inches(5)
height = Inches(4)
# Embed the Excel file as an OLE object
slide.Shapes.AddOLEObject(
Left=left, Top=top, Width=width, Height=height,
ClassName='Excel.Sheet', # Class name for embedding Excel files
FileName=excel_file_path,
LinkToFile=False,
DisplayAsIcon=True # Set to False to display content instead of an icon
)
# Save and close the presentation
presentation.SaveAs(output_ppt_path)
presentation.Close()
app.Quit()
Utilize templates to create slides
If you don’t want to change core document properties but want to change the text and assets within a PowerPoint, you could use templates. This method retains all the original styling and layouts, which can be programmatically customized with new content. Templating can be a good option if you need to:
- Automatically generate monthly or quarterly business review slides that require consistent formatting but updated data.
- Build PowerPoint-based courses that require periodic updates to the material while maintaining a consistent instructional style.
- Quickly adapt a single template to different products or services, customizing content to match target demographics while keeping branding unchanged.
Steps for templating with python-pptx:
- Select a template: Choose an existing PowerPoint file that fits the general structure and style you require. This template will act as the base for all subsequent modifications.
- Modify content: Programmatic alterations allow for dynamic content insertion based on data or business logic, which can significantly streamline the creation of personalized presentations.
- Maintain style integrity: Utilizing a template ensures that all slides adhere to predefined styling rules, enhancing the professional appearance of your presentations.
Example 1: Advanced templating
Here we’re incorporating conditional logic and more complex placeholders, making your template more versatile.
A sample slide with placeholders:
from pptx import Presentation
# Load the existing presentation
presentation = Presentation('template.pptx')
# Dictionary mapping placeholders to their new content
content_map = {
'Placeholder1': 'Title',
'Placeholder2': 'Dynamic content',
# Add more placeholders and content as needed
}
# Iterate through slides and update text based on the content_map
for slide in presentation.slides:
for shape in slide.shapes:
if shape.has_text_frame and shape.text in content_map:
shape.text_frame.text = content_map[shape.text] # Update the text using the mapped value
# Save the modified presentation
presentation.save('updated_output.pptx')
Slide after running the script:
Format paragraphs
The python-pptx library offers immense formatting options to stylize text within your PowerPoint presentations. Here are some essential formatting capabilities that can be achieved with a simple Python script:
- Text alignment: This function allows aligning text either left, right, or center. Additionally, you could adjust it to be justified or distributed evenly across the slide.
- Font customization: You can manipulate text font size, color, type and style, including bold, italics, underlining, and strikethrough.
- Bullet lists: Lists benefit from bullet points or numbering for improved clarity and presentation.
- Indentation and line spacing: Making use of indentation to distinguish paragraphs and manage line spacing to ensure there's sufficient white space improves readability.
- Hyperlinking: Adding hyperlinks to text for directing users to web pages, documents, or email is possible.
- Embedding HTML rich text: There's the capability of embedding HTML-formatted rich text into presentations. While python-pptx does not offer native support for this functionality, developers commonly use a workaround. It involves converting HTML to rtf using external packages like html2rtf, and then embedding the converted rtf as part of the slide.
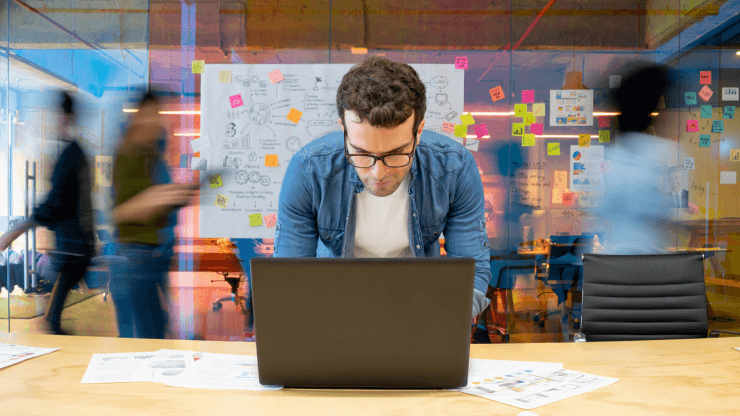
Embed charts in a PowerPoint
Embedding charts into a PowerPoint presentation with Python's python-pptx library can be much faster than building a chart from scratch. When leveraging templates, it can also be an excellent way to systematically update charts with the latest business data. Python-pptx provides a wide range of chart types including column, line, and pie charts. Let’s look at an example.
Example 1: Generate a basic column chart
from pptx import Presentation
from pptx.chart.data import CategoryChartData
from pptx.enum.chart import XL_CHART_TYPE
from pptx.util import Inches
# Create presentation
prs = Presentation()
# Chart data
chart_data = CategoryChartData()
chart_data.categories = ['East', 'West', 'Midwest']
chart_data.add_series('Q1 Sales', (19.2, 21.4, 16.7))
# Add slide
slide_layout = prs.slide_layouts[5]
slide = prs.slides.add_slide(slide_layout)
# Add chart to the slide
x, y, cx, cy = Inches(2), Inches(2), Inches(6), Inches(4.5)
chart = slide.shapes.add_chart(
XL_CHART_TYPE.COLUMN_CLUSTERED, x, y, cx, cy, chart_data
).chart
# Save presentation
prs.save("chart.pptx")
Example 2: Dynamically update chart data
With Python, your chart can dynamically update when your data changes. You can open the presentation and replace the chart data. Rerunning the original script with changed data will overwrite the previous file, which is a viable way of updating chart data.
from pptx import Presentation
from pptx.chart.data import CategoryChartData
# Open presentation
prs = Presentation("chart.pptx")
chart = prs.slides[0].shapes[1].chart
# Chart data
chart_data = CategoryChartData()
chart_data.categories = ['East', 'West', 'Midwest']
chart_data.add_series('Q2 Sales', (15, 10, 25))
# Replace chart data
chart.replace_data(chart_data)
# Save presentation
prs.save("updated_chart.pptx")
Extract information
One of python-pptx's powerful features is information extraction. It allows you to read a pptx file and extract data from the warehouse management system (WMS) such as text, tables, images, or any metadata associated with the document. You can then process data, analyze, modify or export it into a different format such as CSV or plain text.
Example 1: Read and extract data using Pandas
In this example we use Pandas to open the specified Excel document using the Pandas read_excel function, which then reads all the data and prints it. If the document contains multiple sheets, you might want to specify the sheet name or index in the read_excel function.
Example 1: Extract data or text from a PowerPoint presentation (WMS SQL database)
from pptx import Presentation
def extract_text(ppt_file):
ppt = Presentation(ppt_file)
for slide in ppt.slides:
for shape in slide.shapes:
if shape.has_text_frame:
for paragraph in shape.text_frame.paragraphs:
for run in paragraph.runs:
print(run.text)
ppt_file = "example.pptx"
extract_text(ppt_file)
In this simple function, extract_text
, the python-pptx library is used to iterate over each slide in the presentation. From each slide, it checks if any elements (or shapes) contain a text frame. If a text frame is present, the function then retrieves the paragraph text.
PowerPoint automation with SoftKraft
If you’re looking for a development team to bring your document processing vision to life, we’d love to help. We offer Python developers outsourcing that simplify the implementation process, enabling you to achieve business results without the hassle. Our team will guide you in the planning and development of an end-to-end PowerPoint automation solution that perfectly aligns with your business requirements.
Conclusion
Python's ability to seamlessly interface with PowerPoint presentations opens up a vast array of possibilities in terms of automating PowerPoint deck creation, manipulation, and enhancement. With the strategies we’ve outlined in this article, you can take your PowerPoint automation to the next level.